Continued from page 1
Installment 3
PHP Session Handling - Cookies Enabled
Instead of storing session information at
browser through
use of cookies,
information can instead be stored at
server in session files. One session file is created and maintained for each user session. For example, if there are three concurrent users browsing
website, three session files will be created and maintained - one for each user. The session files are deleted if
session is explicitly closed by
PHP script or by a daemon garbage collection process provided by PHP. Good programming practice would call for sessions to be closed explicitly in
script.
The following is a typical server-browser sequence of events that occur when a PHP session handling is used: 1. The server knows that it needs to remember
State of browsing session 2. PHP generates a sssion ID and creates a session file to store future information as required by subsequent pages 3. A cookie is generated wih
session ID at
browser 4. This cookie that stores
session ID is transparently and automatically sent to
server for all subsequent requests to
server
The following PHP session-handling example accomplishes
same outcome as
previous cookie example. Copy
code below (both
php and
html) into a file with
.php extension and test it out.
[?php //starts a session session_start();
//informs PHP that count information needs to be remembered in
session file if (!session_is_registered("count")) { session_register("count"); $count = 0; } else { $count++; }
$session_id = session_id(); ?]
[html] [head] [title]PHP Session Handling - Cookie-Enabled[/title] [/head] [body] The current session id is: [?=$session_id ?] This page has been displayed: [?=$count ?] times. [/body] [/html]
A summary of
functions that PHP provides for session handling are: 1. boolean start_session() - initializes a session 2. string session_id([string id]) - either returns
current session id or specify
session id to be used when
session is created 3. boolean session_register(mixed name [, mixed ...]) - registers variables to be stored in
session file. Each parameter passed in
function is a separate variable 4. boolean session_is_registered(string variable_name) - checks if a variable has been previously registered to be stored in
session file 5. session_unregister(string varriable_name) - unregisters a variable from
session file. Unregistered variables are no longer valid for reference in
session. 6. session_unset() - unsets all session variables. It is important to note that all
variables remain registered. 7. boolean session_destroy() - destroys
session. This is opposite of
start_session function.
The next installment discusses how to manage sessions using PHP session handling functions when cookies are disabled...
Installment 4
PHP Session Handling - Without Cookies
If cookies are disabled at
browser,
above example cannot work. This is because although
session file that stores all
variables is kept at
server, a cookie is still needed at
browser to store
session ID that is used to identify
session and its associated session file. The most common way around this would be to explicitly pass
session ID back to
server from
browser as a query parameter in
URL.
For example,
PHP script generates requests subsequent to
start_session call in
following format: http://www.yourhost.com/yourphpfile.php?PHPSESSID=[actual session ID]
The following are excerpts that illustrate
discussion:
Manually building
URL: $url = "http://www.yoursite.com/yourphppage.php?PHPSESSID=" . session_id(); [a href="[?=$url ?]" rel="nofollow"]Anchor Text[/a]
Building
URL using SID: [a href="http://www.yoursite.com/yourphppage.php?[?=SID ?]" rel="nofollow"]Anchor Text[/a]
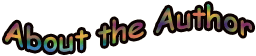
This PHP scripting article is written by John L. John L is the Webmaster of The Ultimate BMW Blog! (http://www.bimmercenter.com).